TMS320C28x
Optimizing C/C++ Compiler
v15.9.0.STS User's Guide
SPRU514 - REVISED SEPTEMBER, 2015
5 Post-Link Optimizer
The TMS320C28x post-link optimizer removes or modifies assembly language instructions to generate better code. The post-link optimizer examines the final addresses of symbols determined by linking and uses this information to make code changes.
Post-link optimization requires the -plink compiler option. The -plink compiler option invokes added passes of the tools that include running the absolute lister and rerunning the assembler and linker. You must use the -plink option following the --run_linker option.
5.1 The Post-Link Optimizer’s Role in the Software Development Flow
The post-link optimizer is not part of the normal development flow. Figure 5-1 shows the flow including the post-link optimizer; this flow occurs only when you use the compiler and the -plink option.
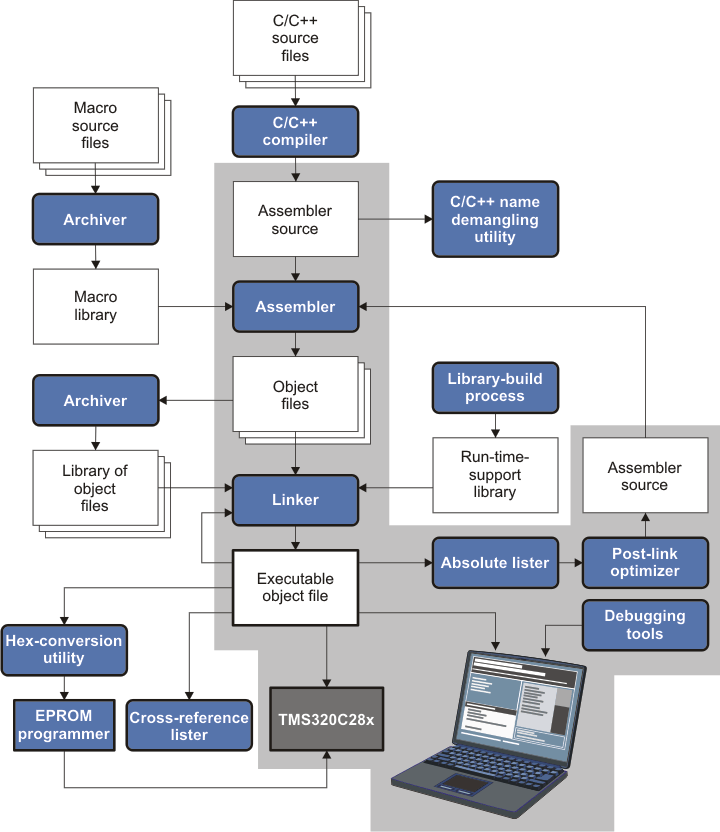
As the flow shows, the absolute lister (abs2000) is also part of the post-link optimizing process. The absolute lister outputs the absolute addresses of all globally defined symbols and COFF sections. The post-link optimizer takes .abs files as input and uses these addresses to perform optimizations. The output is a .pl file, which is an optimized version of the original .asm file. The flow then reruns the assembler and linker to produce a final output file.
The described flow is supported only when you use the compiler (cl2000) and the -plink option. If you use a batch file to invoke each tool individually, you must adapt the flow to use the compiler instead. In addition, you must use the --output_file option to specify an output file name when using the -plink option. See Section 5.8 for more details.
For example, replace these lines:
cl2000 file1.asm file1.obj
cl2000 file2.asm file2.obj
cl2000 --run_linker file1.obj file2.obj lnk.cmd --output_file=prog.out
with this line:
cl2000 file1.asm file2.asm --run_linker lnk.cmd --output_file=prog.out -plink
The advice_only mode is the only post-link optimization support provided for FPU and VCU targets.
5.2 Removing Redundant DP Loads
Post-link optimization reduces the difficulty of managing the Data Page (DP) register by removing redundant DP loads. It does this by tracking the current value of the DP and determining whether the address in a MOV DP,#address instruction is located on the same 64-word page to which the DP is currently pointing. If the address can be accessed using the current DP value, the instruction is redundant and can be removed. For example, consider the following code segment:
MOVZ DP,#name1
ADD @name1,#10
MOVZ DP,#name2
ADD @name2,#10
If name1 and name2 are linked to the same page, the post-link optimizer determines that loading DP with the address of name2 is not necessary, and it comments out the redundant load.
MOVZ DP,#name1
ADD @name1,#10
; <<REDUNDANT>> MOVZ DP,#name2
ADD @name2,#10
This optimization can be used on C files as well. Even though the compiler manages the DP for all global variable references that are defined within a module, it conservatively emits DP loads for any references to global variables that are externally defined. Using the post-link optimizer can help reduce the number of DP loads in these instances.
Additionally, the --map_file linker option can be used to generate a listing of symbols sorted by data page to assist with data layout and DP load reduction. For more information, refer to the Linker Description chapter in the TMS320C28x Assembly Language Tools User's Guide.
5.3 Tracking DP Values Across Branches
In order to track DP values across branches, the post-link optimizer requires that there are no indirect calls or branches, and all possible branch destinations have labels. If an indirect branch or call is encountered, the post-link optimizer will only track the DP value within a basic block. Branch destinations without labels may cause incorrect output from the post-link optimizer.
If the post-link optimizer encounters indirect calls or branches, it issues the following warning:
NO POST LINK OPTIMIZATION DONE ACROSS BRANCHES
Branch/Call must have labeled destination
This warning is issued so that if the file is a hand written assembly file, you can try to change the indirect call/branch to a direct one to obtain the best optimization from the post linker.
5.4 Tracking DP Values Across Function Calls
The post-link optimizer optimizes DP loads after a call to a function if the function is defined in the same file scope. For example, consider the following post-link optimized code:
_main:
LCR #_foo
MOVB AL, #0
;<<REDUNDANT>> MOVZ DP,#_g2
MOV @_g2, #20
LRETR
.global _foo
_foo:
MOVZ DP, #g1
MOV @_g1, #10
LRETR
The MOVZ DP after the function call to _foo is removed by the post-link optimizer as the variables _g1 and _g2 are in the same page and the function _foo already set the DP.
In order for the post-link optimizer to optimize across the function calls, the functions should have only one return statement. If you are running the post-link optimizer on hand written assembly that has more than one return statement per function, the post-link optimization output can be incorrect. You can turn off the optimization across function calls by specifying the -nf option after the -plink option.
5.5 Other Post-Link Optimizations
An externally defined symbol used as a constant operand forces the assembler to choose a 16-bit encoding to hold the immediate value. Since the post-link optimizer has access to the externally defined symbol value, it replaces a 16-bit encoding with an 8-bit encoding when possible. For example:
.ref ext_sym ; externally defined to be 4
:
:
ADD AL, #ext_sym ; assembly will encode ext_sym with 16 bits
Since ext_sym is externally defined, the assembler chooses a 16-bit encoding for ext_sym. The post-link optimizer changes the encoding of ext_sym to an 8-bit encoding:
.ref ext_sym
:
:
; << ADD=>ADDB>> ADD AL,#ext_sym
ADDB AL, #ext_sym
Similarly the post-link optimizer attempts to reduce the following 2-word instructions to 1-word instructions:
2-Word Instructions | 1-Word Instructions |
---|---|
ADD ACC, #imm | ADDB ACC, #imm |
ADD AL, #imm | ADDB AL, #imm |
AND AL, #imm | ANDB AL, #imm |
CMP AL, #imm | CMPB AL, #imm |
MOVL XARn, #imm | MOVB XARn, #imm |
OR AL, #imm | ORB AL, #imm |
SUB ACC, #imm | SUBB ACC, #imm |
SUB AL, #imm | SUBB AL, #imm |
XOR AL, #imm | XORB AL, #imm |
5.6 Controlling Post-Link Optimizations
There are three ways to control post-link optimizations: by excluding files, by inserting specific comments within an assembly file, and by manually editing the post-link optimization file.
5.6.1 Excluding Files (-ex Option)
Specific files can be excluded from the post-link optimization process by using the −ex option. The files to be excluded must follow the −ex option and include file extensions. The −ex option must be used after the −plink option and no other option may follow. For example:
cl2000 file1.asm file2.asm file3.asm --keep_asm --run_linker lnk.cmd -plink -o=prog.out -ex=file3.asm
The file3.asm will be excluded from the post-link optimization process.
5.6.2 Controlling Post-Link Optimization Within an Assembly File
Within an assembly file, post-link optimizations can be disabled or enabled by using two specially formatted comment statements:
;//NOPLINK//
;//PLINK//
Assembly statements following the NOPLINK comment are not optimized. Post-link optimization can be reenabled using the //PLINK// comment.
The PLINK and NOPLINK comment format is not case sensitive. There can be white space between the semicolon and PLINK delimiter. The PLINK and NOPLINK comments must appear on a separate line, alone, and must begin in column 1. For example:
; //PLINK//
5.6.3 Retaining Post-Link Optimizer Output (--keep_asm Option)
The --keep_asm option allows you to retain any post-link files (.pl) and .absolute listing files (.abs) generated by the −plink option. Using the --keep_asm option lets you view any changes the post-link optimizer makes.
The .pl files contain the commented out statement shown with <<REDUNDANT>> or any improvements to instructions, such as <<ADD=>ADDB>>. The .pl files are assembled and linked again to exclude the commented out lines.
5.6.4 Disable Optimization Across Function Calls (-nf Option )
The −nf option disables the post-link optimization across function calls. The post-link optimizer recognizes the function end by the return statement and assumes there is only one return statement per function. In some hand written assembly code, it is possible to have more than one return statement per function. In such cases, the output of the post-link optimization can be incorrect. You can turn off the optimization across function calls by using the− nf option. This option affects all the files.
5.6.5 Annotating Assembly with Advice (--plink_advice_only option)
The --plink_advice_only option annotates assembly code with comments if changes cannot be made safely due to pipeline considerations, such as when float support or VCU support is enabled.
If you use this option, note that the post-link files (.pl), which contain the generated advice, are retained only if you also use the --keep_asm option.
5.7 Restrictions on Using the Post-Link Optimizer
The following restrictions affect post-link optimization:
- The advice_only mode is the only post-link optimization support provided for FPU and VCU targets.
- Branches or calls to unlabeled destinations invalidate DP load optimizations. All branch destinations must have labels.
- If the position of the data sections depends on the size of the code sections, the data page layout information used to decide which DP load instructions to remove may no longer be valid.
For example, consider the following link command file:
SECTIONS
{
.text > MEM,
.mydata > MEM,}
A change in the size of the .text section after optimizing causes the .mydata section to shift. Ensuring that all output data sections are aligned on a 64-word boundary removes this shifting issue. For example, consider the following link command file:
SECTIONS
{
.text > MEM,
.mydata align = 64 > MEM,}
5.8 Naming the Outfile (--output_file Option)
When using the -plink option, you must include the --output_file option. If the output filename is specified in a linker command file, the compiler does not have access to the filename to pass it along to other phases of post-link optimization, and the process will fail. For example:
cl2000 file1.c file2.asm --run_linker --output_file=prog.out lnk.cmd -plink
Because the post-link optimization flow uses the absolute lister, abs2000, it must be included in your path.
Copyright© 2015, Texas Instruments Incorporated. An IMPORTANT NOTICE for this document addresses availability, warranty, changes, use in safety-critical applications, intellectual property matters and other important disclaimers.